Eloquent Test
This tool helps
Laravel
developers learn Eloquent ORM
.
Enter the Eloquent code in the box below and you will get the generated SQL queries and result data.Sample Table
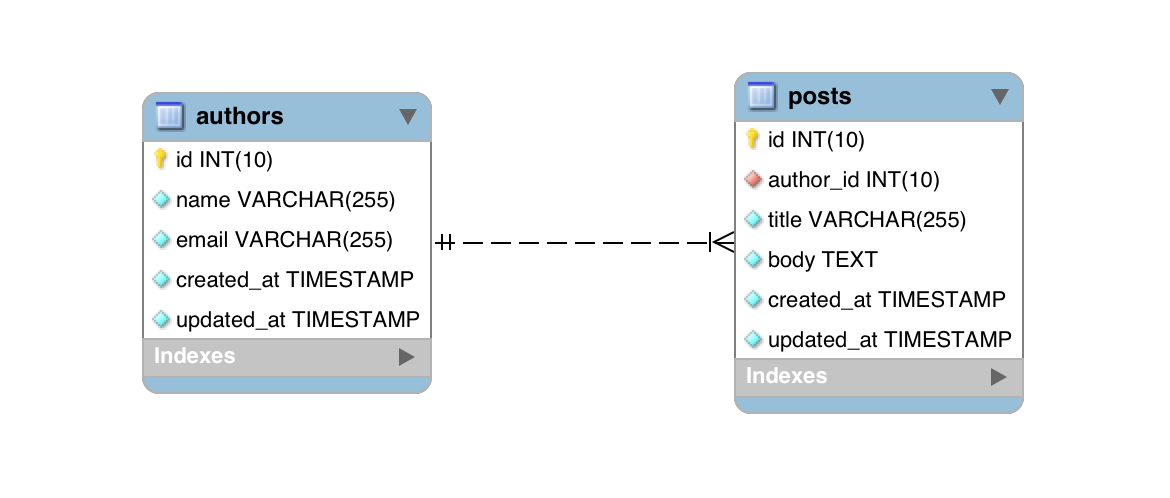
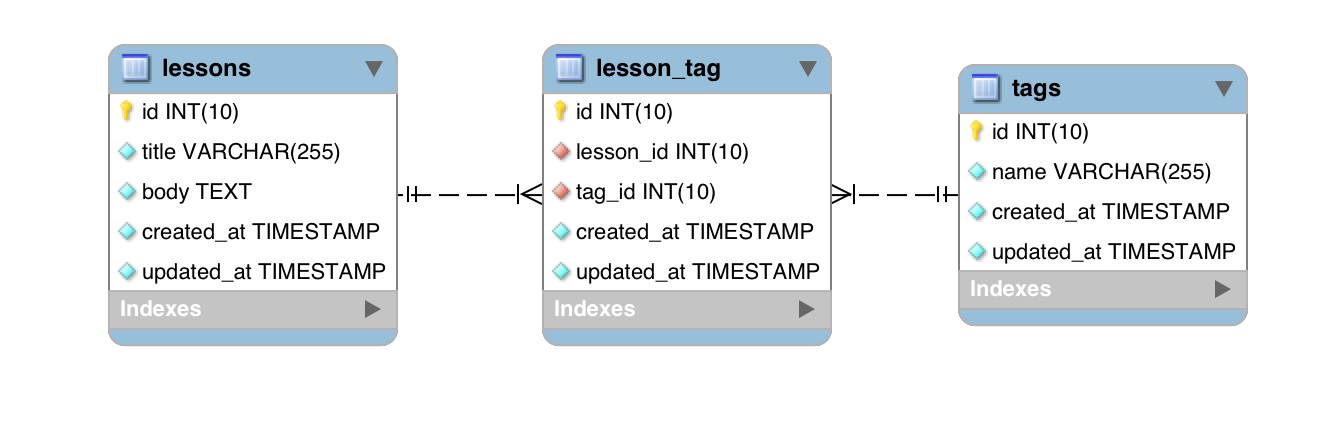
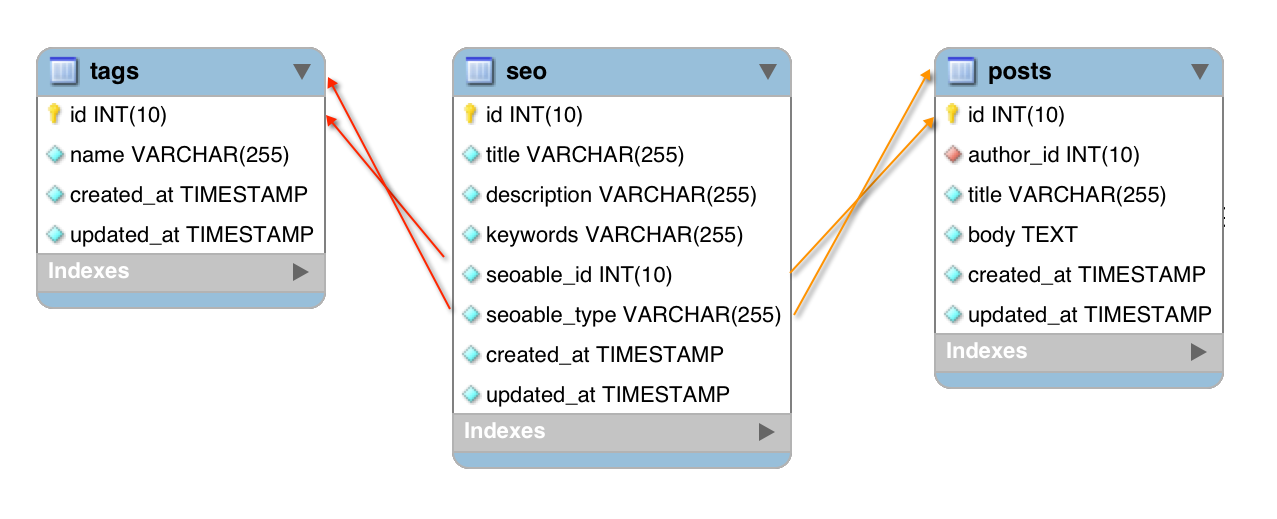
Example Code
Author::find(1)->posts;
- select * from `authors` where `authors`.`id` = '1' limit 1
- select * from `posts` where `posts`.`author_id` = '1'
Author::find(1)->posts()->get();
- select * from `authors` where `authors`.`id` = '1' limit 1
- select * from `posts` where `posts`.`author_id` = '1'
Post::where('title', '=', 'My First Post')->first();
- select * from `posts` where `title` = 'My First Post' limit 1
Post::where('title', 'My First Post')->first();
- select * from `posts` where `title` = 'My First Post' limit 1
Post::whereTitle('My First Post')->first();
- select * from `posts` where `title` = 'My First Post' limit 1
Post::whereTitle('My First Post')->get();
- select * from `posts` where `title` = 'My First Post'
Author::find(1)->posts;
- select * from `authors` where `authors`.`id` = '1' limit 1
- select * from `posts` where `posts`.`author_id` = '1'
Author::with('posts')->find(1);
- select * from `authors` where `authors`.`id` = '1' limit 1
- select * from `posts` where `posts`.`author_id` in ('1')
Author::with(['posts' => function ($query) {
$query->where('title', 'My First Post')->select('title', 'body')->orderBy('created_at', 'DESC');
}])->find(1);
- select * from `authors` where `authors`.`id` = '1' limit 1
- select `title`, `body` from `posts` where `posts`.`author_id` in ('1') and `title` = 'My First Post' order by `created_at` desc
Lesson::find(1)->tags;
- select * from `lessons` where `lessons`.`id` = '1' limit 1
- select `tags`.*, `lesson_tag`.`lesson_id` as `pivot_lesson_id`, `lesson_tag`.`tag_id` as `pivot_tag_id` from `tags` inner join `lesson_tag` on `tags`.`id` = `lesson_tag`.`tag_id` where `lesson_tag`.`lesson_id` = '1'
Tag::join('lesson_tag', 'lesson_tag.tag_id', '=', 'tags.id')
->groupBy('tags.name')
->get(['tags.name', DB::raw('count(*) as lessons_count')]);
- select `tags`.`name`, count(*) as lessons_count from `tags` inner join `lesson_tag` on `lesson_tag`.`tag_id` = `tags`.`id` group by `tags`.`name`
$posts = Post::whereAuthorId(1)->get();
foreach ($posts as $post) {
$name = $post->author->name;
}
- select * from `posts` where `author_id` = '1'
$posts = Post::with('author')->whereAuthorId(1)->get();
foreach ($posts as $post) {
$name = $post->author->name;
}
- select * from `posts` where `author_id` = '1'
- select * from `authors` where `authors`.`id` in ('1')
Author::find(1)->posts->count();
- select * from `authors` where `authors`.`id` = '1' limit 1
- select * from `posts` where `posts`.`author_id` = '1'
Author::find(1)->posts()->count();
- select * from `authors` where `authors`.`id` = '1' limit 1
- select count(*) as aggregate from `posts` where `posts`.`author_id` = '1'
Author::find(1)->posts()->get()->count();
- select * from `authors` where `authors`.`id` = '1' limit 1
- select * from `posts` where `posts`.`author_id` = '1'
Post::orderBy('created_at', 'DESC')->get();
- select * from `posts` order by `created_at` desc
Post::latest()->get();
- select * from `posts` order by `created_at` desc
Post::orderBy('created_at', 'ASC')->get();
- select * from `posts` order by `created_at` asc
Post::oldest()->get();
- select * from `posts` order by `created_at` asc
Post::find(1)->seo->first();
- select * from `posts` where `posts`.`id` = '1' limit 1
- select * from `seo` where `seo`.`seoable_id` = '1' and `seo`.`seoable_type` = 'Post'
Post::with('seo')->find(1);
- select * from `posts` where `posts`.`id` = '1' limit 1
- select * from `seo` where `seo`.`seoable_id` in ('1') and `seo`.`seoable_type` = 'Post'
Seo::find(1)->seoable;
- select * from `seo` where `seo`.`id` = '1' limit 1
- select * from `posts` where `posts`.`id` = '1' limit 1
Seo::with('seoable')->get();
- select * from `seo`
- select * from `posts` where `id` in ('1', '2')
- select * from `tags` where `id` in ('1')